A Simplified Snapping Module for Mapbox GL JS

- Published on
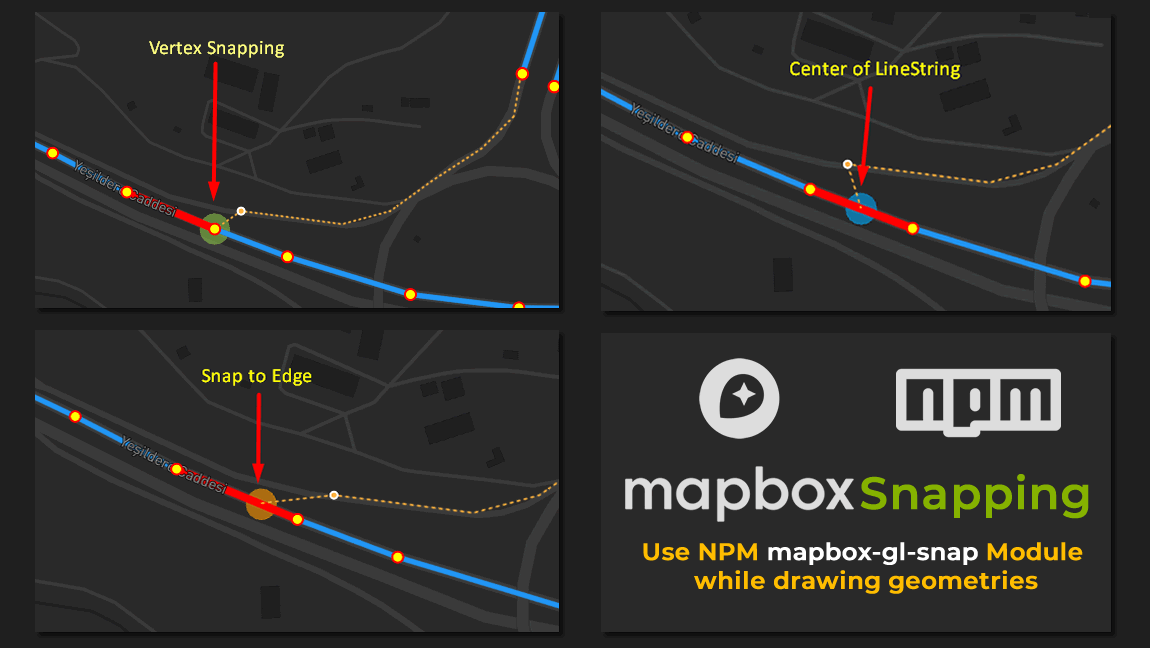
Article Number : 23
Introducing MapboxSnap: A Simplified Snapping Module for Mapbox GL JS
As a developer working extensively with map-based applications, I noticed a common challenge when users were trying to draw precise geometries on a map. The need for an intuitive snapping mechanism that allowed for accurate placements of points, lines, and edges became evident. That’s why I developed MapboxSnap, a utility designed to make drawing on maps easier and more accurate by enabling snapping to specific geometric points, edges, or midpoints.
Why I Built MapboxSnap
In many GIS and mapping applications, precision is key. Users often struggle with drawing geometries that perfectly align with existing features, especially when working with complex maps that require high accuracy. MapboxSnap was created to address this challenge, offering a streamlined and user-friendly solution to snap to nearby features with ease. Whether you’re aligning to a vertex, a line’s midpoint, or along an edge, MapboxSnap makes the process effortless, enhancing both the user experience and the accuracy of the drawn geometries.
Key Features of MapboxSnap
Layer-Based Snapping: Snap to specific geometries within defined layers, ensuring that snapping is restricted to relevant features only.
Rule-Based Snapping: Define the snapping rules you need. Choose whether to snap to vertices, midpoints, or edges, and in which order these rules should apply.
Customizable Snap Radius: Control how close the cursor needs to be to a feature for snapping to occur by adjusting the snap radius.
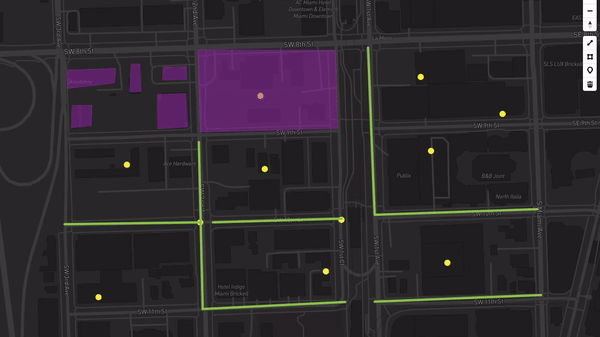
How to Use MapboxSnap
Installation
To get started, you’ll need to install the necessary libraries:
npm install mapbox-gl @mapbox/mapbox-gl-draw @turf/turf mapbox-gl-snap
Importing the Library
Depending on your project setup, you can import the library using either PureJS, ES Modules or CommonJS:
Browser PureJS:
<script src="https://cdn.jsdelivr.net/gh/gislayer/mapbox-gl-snap/dist/purejs/mapbox-gl-snap.js"></script>
ES Modules:
import MapboxSnap from 'mapbox-gl-snap/dist/cjs/MapboxSnap';
CommonJS:
const MapboxSnap = require('mapbox-gl-snap/dist/esm/MapboxSnap');
Example Usage
Here’s a basic example of how to implement MapboxSnap in your project:
import mapboxgl from 'mapbox-gl';
import MapboxDraw from '@mapbox/mapbox-gl-draw';
import MapboxSnap from 'mapbox-gl-snap';
const map = new mapboxgl.Map({
container: 'map',
style: 'mapbox://styles/mapbox/dark-v11',
center: [0, 0],
zoom: 16,
});
map.on('style.load', () => {
const drawing = new MapboxDraw({
displayControlsDefault: false,
controls: {
polygon: true,
line_string: true,
point: true,
trash: true,
},
});
map.addControl(drawing);
// Initialize MapboxSnap
const mapboxSnap = new MapboxSnap({
map: map,
drawing: drawing,
options: {
layers: ['layer1', 'layer2'], // Specify layers to snap to
radius: 15, // Snap radius in pixels
rules: ['vertex', 'midpoint', 'edge'] // Snapping rules
},
});
});
Visualizing the Snap Process
Here are some visuals demonstrating the snapping behavior:
- Vertex Snapping: When you hover near a vertex, the radius circle turns green, indicating a snap to a vertex point.
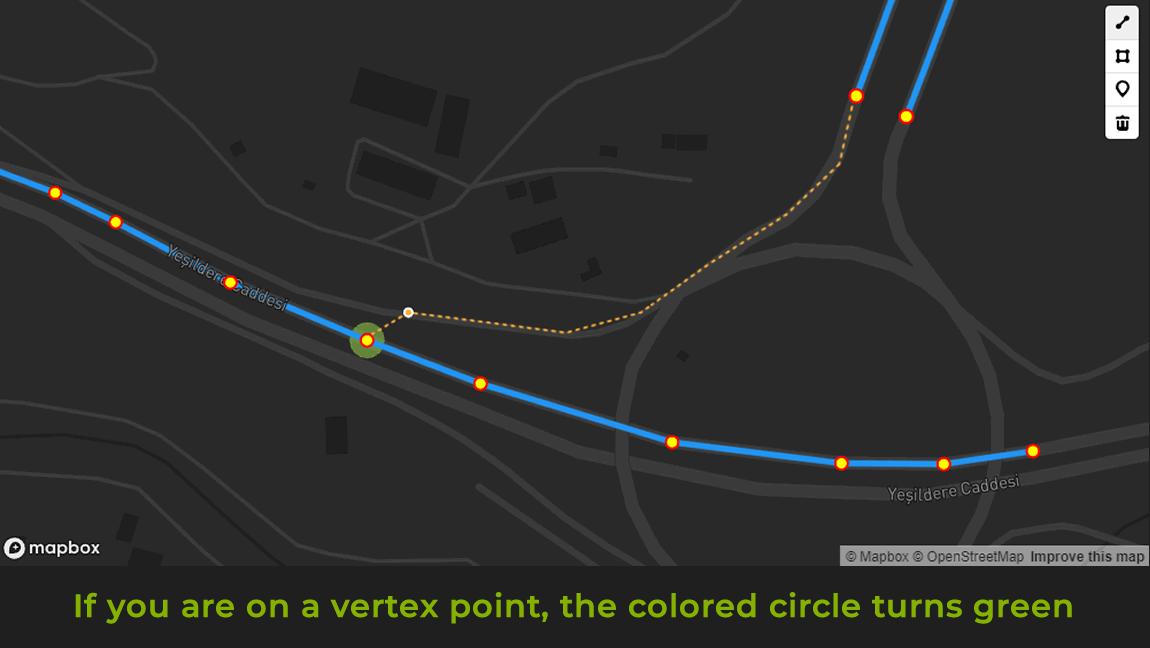
- Midpoint Snapping: When hovering over the midpoint of a line, the radius circle turns blue, signifying a snap to the midpoint.
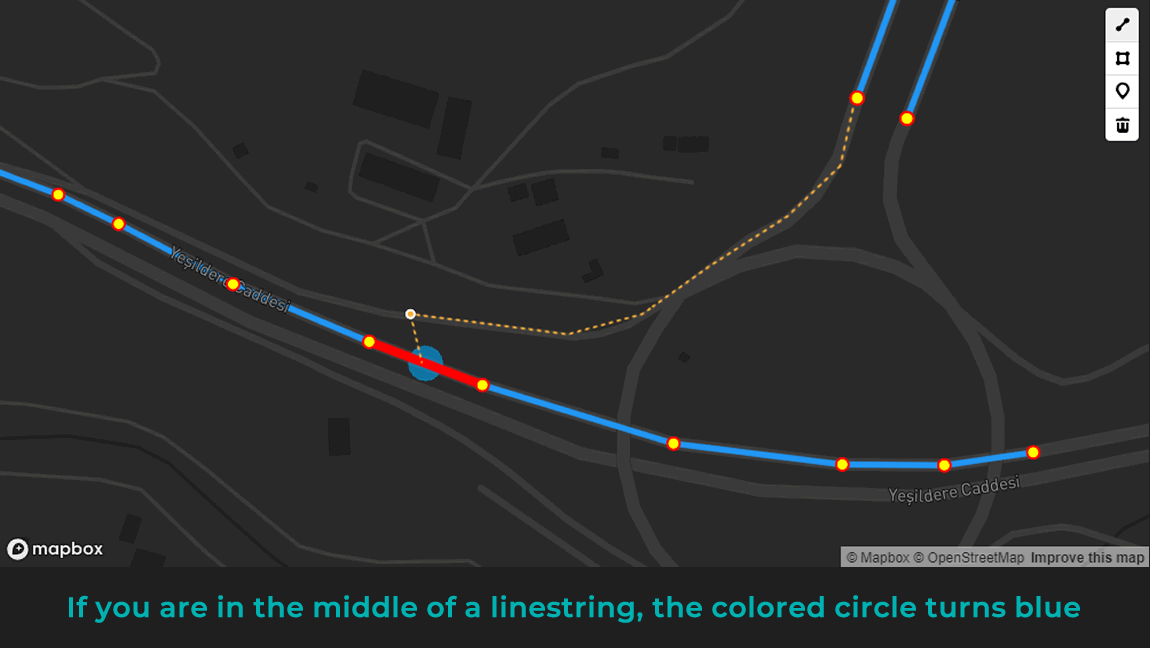
- Edge Snapping: When near an edge of a linestring or polygon, the radius circle turns orange, indicating a snap to the edge.
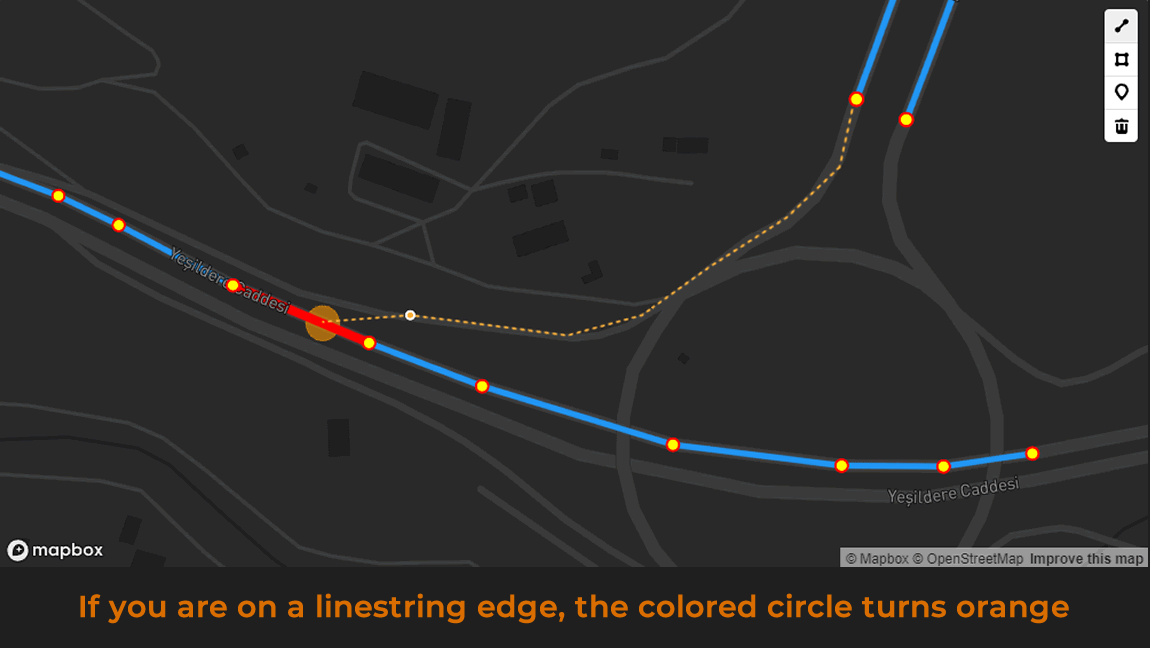
Conclusion
MapboxSnap is a powerful addition to any Mapbox GL JS-based application that requires precise drawing capabilities. By allowing users to snap to vertices, midpoints, and edges with customizable rules and radius settings, it significantly enhances the accuracy and usability of map-based tools.
Whether you are building a GIS application, a map editor, or any project that involves drawing geometries on a map, MapboxSnap will streamline your workflow and improve the end-user experience.
Keywords
- Mapbox GL JS
- Map Snapping
- Geometric Precision
- Vertex Snapping
- Midpoint Snapping
- Edge Snapping
- Map Drawing Tools
- TurfJS
- GeoJSON
By integrating MapboxSnap into your projects, you'll be able to offer your users a more intuitive and precise drawing experience. I hope this tool helps you as much as it has helped in my projects!